6502 Emulator Mac
This is my C++ emulator of the MOS Technology 6502 CPU. The code emulates a fully functional 6502 CPU and it seems to be pretty fast too. Some minor tricks have been introduced to greatly reduce the overall execution time.
Nox Archaist is a new role playing game in development by 6502 Workshop exclusively for the Apple II platform and emulators, with floppy a. Blog Archive 2021 (5). For Mac OSX user 65xxTools have been successfully tested on VMware Fusion. NOTE: This software appends values to the PATH Environment Variable in Windows. This is for the directory paths for executable. Windows has a limit of 8192 characters for the PATH.
Interrupt and bus read/write operations are emulated as well.
Github repo: https://github.com/gianlucag/mos6502
Andy is the best Android Emulator for PC or Mac. It lets you connect your Android device and desktop together in a virtual environment to provide an ideal gaming experience. The application is. Download Mac Emulator: Mini vMac (A fully functional Mac Plus emulator for the Android platform) and many other apps. Commodore VC20-Emulator (6502, VIC, Display, Tastatur) als AVR-Studio Assembler-Projekt. ATMega based home computer - ATMega162 emuliert 6502 mit SCART.
What’s a 6502???

Here a brief descrption: http://en.wikipedia.org/wiki/MOS_Technology_6502
Main features:
- 100% coverage of legal opcodes
- decimal mode implemented
- read/write bus callback
- jump table opcode selection
Still to implement
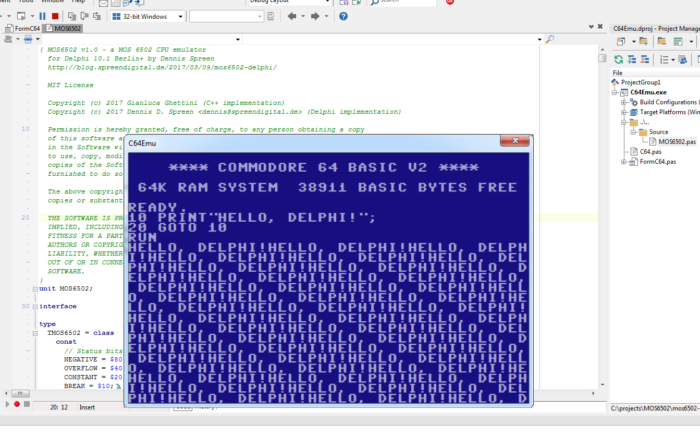
- 100% cycle accuracy
- illegal opcodes
- hardware glitches, the known ones of course 🙂
The emulator was extensively tested against this test suite:
and in parallel emulation with fake6502 http://rubbermallet.org/fake6502.c
so expect nearly 100% compliance with the real deal… at least on the normal behavior: as I said stuff like illegal opcodes or hardware glitches are currently not implemented.
Why yet another 6502 emulator?
Just for fun :). This CPU (and its many derivatives) powered machines such as:
- Apple II
- Nintendo Entertainment system (NES)
- Atari 2600
- Commodore 64
- BBC micro
and many other embedded devices still used today. You can use this emulator in your machine emulator project. However cycle accuracy is not yet implemented so mid-frame register update tricks cannot be reliably emulated.
Some theory behind emulators: emulator types
You can group all the CPU emulators out there in 4 main categories:
- switch-case based
- jump-table based
- PLA or microcode emulation based
- graph based
Graph based emulators are the most accurate as they emulate the connections between transistors inside the die of the CPU. They emulate even the unwanted glitches, known and still unknown. However the complexity of such emulators is non-linear with the number of transistors: in other word, you don’t want to emulate a modern Intel quad core using this approach!!!
for an example check this out: http://visual6502.org/JSSim/index.html

The PLA/microcode based are the best as they offer both speed and limited complexity. The switch-case based are the simpler ones but also the slowest: the opcode value is thrown inside a huge switch case which selects the code snippet to execute; compilers can optimize switch case to reach near O(log(n)) complexity but they hardly do it when dealing with sparse integers (like most of the CPU opcode tables).
Emulator features
My project is a simple jump-table based emulator: the actual value of the opcode (let’s say 0x80) is used to address a function pointer table, each entry of such table is a C++ function which emulates the behavior of the corresponding real instruction.
All the 13 addressing modes are emulated:

All the 151 opcodes are emulated. Since the 6502 CPU uses 8 bit to encode the opcode value it also has a lot of “illegal opcodes” (i.e. opcode values other than the designed 151). Such opcodes perform weird operations, write multiple registers at the same time, sometimes are the combination of two or more “valid” opcodes. Such illegals were used to enforce software copy protection or to discover the exact CPU type.
The illegals are not supported yet, so instead a simple NOP is executed.
Inner main loop
It’s a classic fetch-decode-execute loop:
6502 Emulator Mac Os
The next instruction (the opcode value) is retrieved from memory. Then it’s decoded (i.e. the opcode is used to address the instruction table) and the resulting code block is executed.
Public methods
The emulator comes as a single C++ class with five public methods:
- mos6502(BusRead r, BusWrite w);
- void NMI();
- void IRQ();
- void Reset();
- void Run(uint32_t n);
mos6502(BusRead r, BusWrite w);
it’s the class constructor. It requires you to pass two external functions:

uint8_t MemoryRead(uint16_t address);
void MemoryWrite(uint16_t address, uint8_t value);
respectively to read/write from/to a memory location (16 bit address, 8 bit value). In such functions you can define your address decoding logic (if any) to address memory mapped I/O, external virtual devices and such.
void NMI();
triggers a Non-Mascherable Interrupt request, as done by the external pin of the real chip
void IRQ();
triggers an Interrupt ReQuest?, as done by the external pin of the real chip
void Reset();
performs an hardware reset, as done by the external pin of the real chip
void Run(uint32_t n);
It runs the CPU for the next ‘n’ machine instructions.
Links
Some useful stuff I used…
|